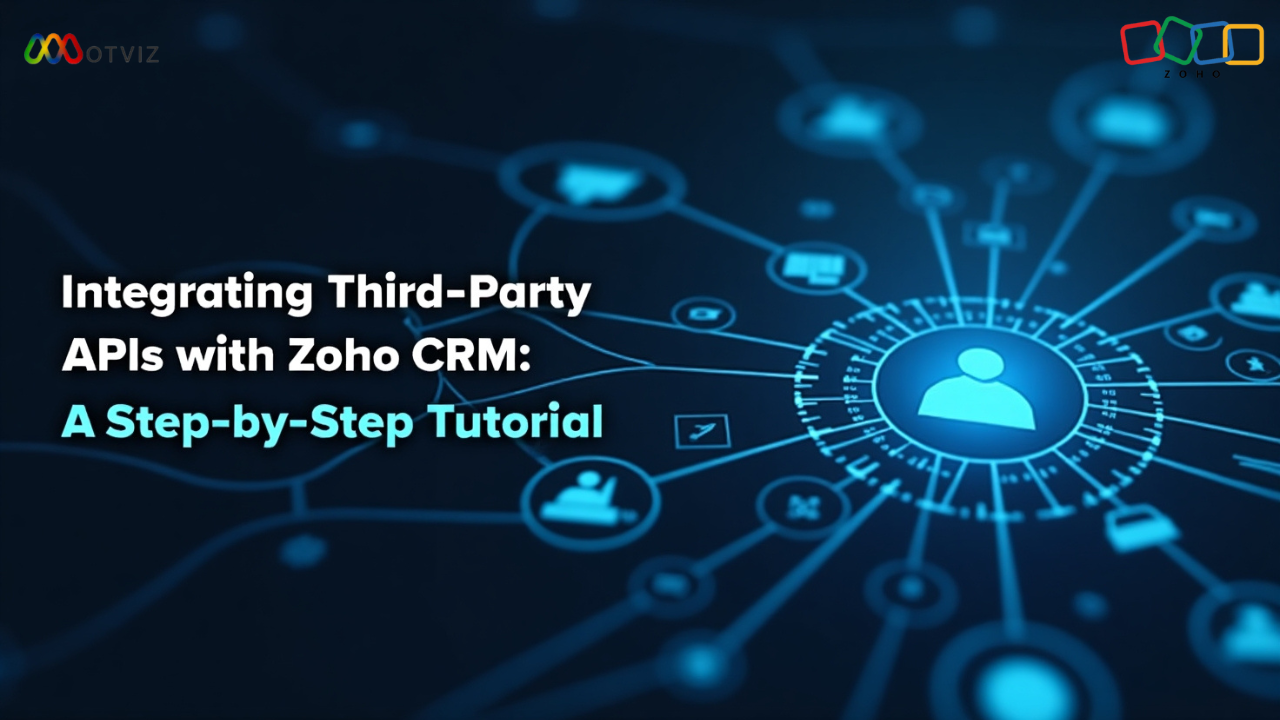
Integrating Third-Party APIs with Zoho CRM: A Step-by-Step Tutorial
Zoho CRM is a powerful platform that enables businesses to manage customer relationships effectively. One of its main strengths is the flexibility of integration with third-party applications, allowing companies to optimize processes and increase productivity. In this tutorial, we will guide you through the process of integrating third-party APIs with Zoho CRM, from authentication to data synchronization.
Prerequisites
Before you begin, make sure you have the following:
- Zoho CRM Account: A Zoho CRM account with admin access.
- API Documentation: Detailed API documentation of the third-party application you want to integrate.
- Programming knowledge: Basic understanding of programming concepts and API communication (e.g. RESTful APIs).
- Development environment: Tools like Postman for testing APIs and a code editor for writing scripts.
Step 1: Understanding the Zoho CRM API Structure
Zoho CRM provides a comprehensive API framework that supports various modules such as Leads, Contacts, Deals, and Custom Modules. Familiarize yourself with the following:
- Authentication: Zoho CRM uses OAuth 2.0 for authentication. This ensures secure communication between Zoho CRM and third-party applications.
- API Endpoints: Explore the API documentation to understand the endpoints, request methods (GET, POST, PUT, DELETE), and required parameters.
- Rate Limits: Zoho imposes rate limits on API usage, so plan your API calls accordingly.
Step 2: Configure OAuth authentication
Authentication is a critical step to establish a secure connection between Zoho CRM and the third-party application. Follow these steps:
- Create a client:
- Log in to the Zoho API Console.
- Create a new client and select the appropriate client type (e.g. server-based or self-client).
- Note the Client ID and Client Secret.
- Generate authorization code:
- Construct the authorization URL using the Zoho OAuth endpoint.
- Access the URL in your browser to log in and authorize the application.
- Copy the authorization code from the redirected URL.
- Exchange authorization code for access token:
- Use a tool like Postman to send a POST request to the Zoho token endpoint.
- Include parameters such as Client ID, Client Secret, Grant Type, and Redirect URI.
- Receive an access token and a refresh token for API communication.
Step 3: Test API endpoints with Postman
Before integrating APIs into your code, test the endpoints using Postman to validate the request and response.
- Setting headers:
- Add Authorization: Zoho-oauthtoken <access_token>.
- Set Content-Type: application/json for JSON data.
- Send API requests:
- Test the required endpoints of Zoho CRM and third-party application.
- Validate response codes and data structure.
- Debugging errors:
- Check for error messages and make sure all required parameters are included.
Step 4: Write the integration code
Once you have validated the APIs, start writing code to integrate Zoho CRM with your third-party application.
Example: PHP script for lead integration
Here is an example PHP script to send lead data from a third-party application to Zoho CRM:
<? php
$accessToken = ‘your_access_token’;
$url = ‘https://www.zohoapis.com/crm/v2/Leads‘;
$data = [
‘data’ => [
[
‘First_Name’ => ‘John’,
‘Last Name’ => ‘Doe’,
‘Email’ => ‘john.doe@example.com‘,
‘Phone’ => ‘1234567890’,
]
]
];
$options = [
‘http’ => [
‘header’ => [
‘Authorization: Zoho-oauthtoken ‘ . $accessToken,
‘Content-Type: application/json’,
],
‘method’ => ‘POST’,
‘content’ => json_encode($data),
]
];
$context = stream_context_create($options);
$response = file_get_contents($url, false, $context);
if ($answer === FALSE) {
die(‘An error occurred’);
}
echo $response;
?>
Key Points:
- Replace your_access_token with a valid access token.
- Use the correct endpoint for the desired module.
Step 5: Schedule regular data synchronization
To keep the data up-to-date, implement periodic synchronization between Zoho CRM and the third-party application. Use a scheduler like CRON (for Linux) or Task Scheduler (for Windows) to automate API calls.
Example: CRON Task
Add the following line to your crontab file to run the script every hour:
0 * * * * php /path/to/your/script.php